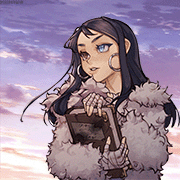
страницы
Сообщений 1 страница 3 из 3
Поделиться12024-11-04 15:11:22
статы и инфа под мобилу
[html]
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Character Card</title>
<style>
.character-card {
display: flex;
flex-direction: column;
width: 100%;
background-color: var(--white);
border-radius: 6px;
border: 1px solid var(--blackmedium);
padding: 20px;
font-family: Arial, sans-serif;
box-sizing: border-box;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
}
.character-header {
font-size: 24px;
color: var(--amscolor);
margin-bottom: 20px;
text-align: center;
font-weight: bold;
width: 100%;
}
.character-content {
display: flex;
flex-direction: column;
align-items: center;
}
.chart-container {
width: 100%;
max-width: 300px;
height: 300px;
margin-bottom: 20px;
}
.character-stats {
width: 100%;
text-align: left;
}
.character-stats p {
margin: 5px 0;
font-size: 1rem;
color: var(--black);
}
@media (min-width: 600px) {
.character-content {
flex-direction: row;
justify-content: space-between;
align-items: flex-start;
}
.chart-container {
width: 50%;
margin-bottom: 0;
}
.character-stats {
width: 45%;
padding-left: 20px;
}
}
</style>
</head>
<body>
<div class="character-card">
<div class="character-header">Информация о персонаже</div>
<div class="character-content">
<div class="chart-container">
<canvas id="spiderChart" width="300" height="300"></canvas>
</div>
<div class="character-stats">
<p><b>Имя Клан:</b> Имя и Клан</p>
<p><b>Возраст:</b> возраст</p>
<p><b>Специализация:</b> медицина, ниндзя сенсор и т.д.</p>
<p><b>Ранг:</b> генин, чунин, джонин</p>
<p><b>Возраст окончания академии:</b> возраст</p>
<p><b>Возраст становления чунином:</b> возраст</p>
<p><b>Участие в экзаменах:</b> 7</p>
</div>
</div>
</div>
<script>
const canvas = document.getElementById('spiderChart');
const ctx = canvas.getContext('2d');
const labels = ['Нин', 'Тай', 'Ген', 'Инт', 'Сил', 'Ско', 'Чак', 'Печ'];
const data = [0.5, 1.5, 2, 2.5, 3, 4, 4.5, 2]; // Пример данных
const drawSpiderChart = (ctx, labels, data, maxValue) => {
ctx.clearRect(0, 0, canvas.width, canvas.height);
const centerX = canvas.width / 2;
const centerY = canvas.height / 2;
const radius = 100;
const backgroundRadius = 120;
const angleStep = (2 * Math.PI) / labels.length;
const labelOffset = 40;
const gradient = ctx.createRadialGradient(centerX, centerY, 0, centerX, centerY, backgroundRadius);
gradient.addColorStop(0, 'rgba(255, 180, 0, 1)');
gradient.addColorStop(0.7, 'rgba(255, 100, 0, 1)');
gradient.addColorStop(1, 'rgba(255, 40, 0, 1)');
ctx.beginPath();
ctx.arc(centerX, centerY, backgroundRadius, 0, Math.PI * 2);
ctx.closePath();
ctx.fillStyle = gradient;
ctx.fill();
ctx.strokeStyle = '#000';
ctx.lineWidth = 1;
for (let i = 1; i <= 5; i++) {
ctx.beginPath();
const r = (radius / 5) * i;
for (let j = 0; j <= labels.length; j++) {
const x = centerX + r * Math.cos(angleStep * j - Math.PI / 2);
const y = centerY + r * Math.sin(angleStep * j - Math.PI / 2);
j === 0 ? ctx.moveTo(x, y) : ctx.lineTo(x, y);
}
ctx.closePath();
ctx.stroke();
}
for (let i = 0; i < labels.length; i++) {
const x = centerX + radius * Math.cos(angleStep * i - Math.PI / 2);
const y = centerY + radius * Math.sin(angleStep * i - Math.PI / 2);
ctx.beginPath();
ctx.moveTo(centerX, centerY);
ctx.lineTo(x, y);
ctx.stroke();
}
ctx.font = 'bold 12px Arial';
ctx.fillStyle = '#333';
for (let i = 0; i < labels.length; i++) {
const x = centerX + radius * Math.cos(angleStep * i - Math.PI / 2);
const y = centerY + radius * Math.sin(angleStep * i - Math.PI / 2);
const labelX = x + Math.cos(angleStep * i - Math.PI / 2) * labelOffset;
const labelY = y + Math.sin(angleStep * i - Math.PI / 2) * labelOffset;
ctx.textAlign = 'center';
ctx.textBaseline = 'middle';
ctx.fillText(labels[i], labelX, labelY);
}
ctx.beginPath();
ctx.fillStyle = 'rgba(255, 255, 255, 0.5)';
ctx.strokeStyle = '#000';
for (let i = 0; i < data.length; i++) {
const value = (data[i] / maxValue) * radius;
const x = centerX + value * Math.cos(angleStep * i - Math.PI / 2);
const y = centerY + value * Math.sin(angleStep * i - Math.PI / 2);
i === 0 ? ctx.moveTo(x, y) : ctx.lineTo(x, y);
}
ctx.closePath();
ctx.fill();
ctx.stroke();
};
drawSpiderChart(ctx, labels, data, 5);
</script>
</body>
</html>
[/html]
Поделиться22024-11-09 02:32:03
ачивки один
[html]
<style>
/* Основные стили для карточки */
.award-card {
align-items: flex-start;
width: 100%;
background-color: var(--white);
border-radius: 6px;
border: 1px solid var(--blackmedium);
padding: 20px;
font-family: Arial, sans-serif;
box-sizing: border-box;
}
.award-card h2 {
text-align: center;
font-size: 18px;
margin-bottom: 10px;
color: #555;
}
/* Стили для списка наград */
.awards {
display: flex;
gap: 4px;
justify-content: center;
margin-top: 10px;
flex-wrap: wrap;
}
.award-item {
position: relative;
display: flex;
align-items: center;
justify-content: center;
width: 50px;
height: 50px;
background-color: #ffffff;
border-radius: 4px;
box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.05);
border: 1px solid #e0e0e0;
cursor: pointer;
}
/* Стили для изображения */
.award-icon {
width: 50px;
height: 50px;
transition: transform 0.3s ease;
object-fit: cover;
}
/* Эффект увеличения при наведении только на картинку */
.award-item:hover .award-icon {
transform: scale(1.2);
}
/* Стили для всплывающего описания */
.award-item:hover .tooltip {
opacity: 1;
visibility: visible;
}
.tooltip {
position: absolute;
top: -100%;
left: 50%;
transform: translateX(-50%);
background-color: #333;
color: #fff;
padding: 5px 10px;
border-radius: 4px;
font-size: 12px;
white-space: nowrap;
opacity: 0;
visibility: hidden;
transition: opacity 0.3s;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.2);
z-index: 1;
}
.tooltip::after {
content: "";
position: absolute;
bottom: -5px;
left: 50%;
transform: translateX(-50%);
border-width: 5px;
border-style: solid;
border-color: #333 transparent transparent transparent;
}
/* Адаптивные стили для мобильных устройств */
@media (max-width: 600px) {
.awards {
grid-template-columns: 1fr 1fr;
}
.award-card {
padding: 10px;
}
.award-item {
padding: 5px;
}
.tooltip {
font-size: 10px; /* Уменьшаем размер текста в тултипе */
padding: 3px 8px; /* Уменьшаем отступы тултипа */
max-width: 100px; /* Ограничиваем максимальную ширину тултипа */
white-space: normal; /* Разрешаем перенос текста на следующую строку */
word-wrap: break-word; /* Переносим слова, если они выходят за границы */
}
}
</style>
</head>
<body>
<div class="award-card">
<h2>Внеигровые награды</h2>
<div class="awards">
<!-- Первый ряд -->
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/44576.png" alt="Медаль за храбрость" class="award-icon">
<div class="tooltip">Медаль за храбрость</div>
</div>
<div class="award-item">
<img src="https://forumupload.ru/uploads/0013/66/99/2/90257.png" alt="Орден чести" class="award-icon">
<div class="tooltip">Орден чести</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/97862.png" alt="Знак отличия" class="award-icon">
<div class="tooltip">Знак отличия</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://static.wikia.nocookie.net/fortnite/images/5/50/Scroll_-_Back_Bling_-_Fortnite.png" alt="Приз зрительских симпатий" class="award-icon">
<div class="tooltip">Приз зрительских симпатий</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/44576.png" alt="Медаль за храбрость" class="award-icon">
<div class="tooltip">Медаль за храбрость</div>
</div>
<div class="award-item">
<img src="https://forumupload.ru/uploads/0013/66/99/2/90257.png" alt="Орден чести" class="award-icon">
<div class="tooltip">Орден чести</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/97862.png" alt="Знак отличия" class="award-icon">
<div class="tooltip">Знак отличия</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://static.wikia.nocookie.net/fortnite/images/5/50/Scroll_-_Back_Bling_-_Fortnite.png" alt="Приз зрительских симпатий" class="award-icon">
<div class="tooltip">Приз зрительских симпатий</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/44576.png" alt="Медаль за храбрость" class="award-icon">
<div class="tooltip">Медаль за храбрость</div>
</div>
<div class="award-item">
<img src="https://forumupload.ru/uploads/0013/66/99/2/90257.png" alt="Орден чести" class="award-icon">
<div class="tooltip">Орден чести</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/97862.png" alt="Знак отличия" class="award-icon">
<div class="tooltip">Знак отличия</div>
</div>
<!-- второй ряд -->
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://static.wikia.nocookie.net/fortnite/images/5/50/Scroll_-_Back_Bling_-_Fortnite.png" alt="Приз зрительских симпатий" class="award-icon">
<div class="tooltip">Приз зрительских симпатий</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/44576.png" alt="Медаль за храбрость" class="award-icon">
<div class="tooltip">Медаль за храбрость</div>
</div>
<div class="award-item">
<img src="https://forumupload.ru/uploads/0013/66/99/2/90257.png" alt="Орден чести" class="award-icon">
<div class="tooltip">Орден чести</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/97862.png" alt="Знак отличия" class="award-icon">
<div class="tooltip">Знак отличия</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://static.wikia.nocookie.net/fortnite/images/5/50/Scroll_-_Back_Bling_-_Fortnite.png" alt="Приз зрительских симпатий" class="award-icon">
<div class="tooltip">Приз зрительских симпатий</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
<div class="award-item">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
<div class="tooltip">Почетная грамота</div>
</div>
</div>
</div>
</body>
</html>
[/html]
Поделиться32024-12-02 19:17:41
ачивки два
[html]
<style>
/* Основные стили для карточки */
.award-card {
align-items: flex-start;
width: 100%;
background-color: var(--white);
border-radius: 6px;
border: 1px solid var(--blackmedium);
padding: 20px;
font-family: Arial, sans-serif;
box-sizing: border-box;
}
.award-card h2 {
text-align: center;
font-size: 18px;
margin-bottom: 10px;
color: #555;
}
/* Стили для списка наград */
.awards {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
gap: 8px;
margin-top: 10px;
}
.award-item {
position: relative;
display: flex;
align-items: center;
background-color: #ffffff;
border-radius: 4px;
padding: 5px;
box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.05);
border: 1px solid #e0e0e0;
font-size: 12px;
cursor: pointer;
}
/* Стили для изображения и текста награды */
.award-icon-container {
width: 50px;
height: 50px;
overflow: visible; /* Позволяет изображению выходить за пределы контейнера */
border-radius: 4px;
border: 0px solid #ddd;
margin-right: 8px;
display: flex;
align-items: center;
justify-content: center;
position: relative;
}
.award-icon {
width: 100%;
height: 100%;
transition: transform 0.3s ease;
object-fit: cover;
}
/* Эффект увеличения при наведении только на картинку */
.award-icon-container:hover .award-icon {
transform: scale(1.2);
}
.award-name {
flex-grow: 1;
color: #333;
font-weight: bold;
}
/* Стили для всплывающего описания */
.award-item:hover .tooltip {
opacity: 1;
visibility: visible;
}
.tooltip {
position: absolute;
top: -100%;
left: 50%;
transform: translateX(-50%);
background-color: #333;
color: #fff;
padding: 5px 10px;
border-radius: 4px;
font-size: 12px;
white-space: nowrap;
opacity: 0;
visibility: hidden;
transition: opacity 0.3s;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.2);
z-index: 1;
}
.tooltip::after {
content: "";
position: absolute;
bottom: -5px;
left: 50%;
transform: translateX(-50%);
border-width: 5px;
border-style: solid;
border-color: #333 transparent transparent transparent;
}
/* Адаптивные стили для мобильных устройств */
@media (max-width: 600px) {
.awards {
grid-template-columns: 1fr 1fr;
}
.award-card {
padding: 10px;
}
.award-item {
padding: 5px;
}
.tooltip {
font-size: 10px; /* Уменьшаем размер текста в тултипе */
padding: 3px 8px; /* Уменьшаем отступы тултипа */
max-width: 100%; /* Ограничиваем максимальную ширину тултипа */
white-space: normal; /* Разрешаем перенос текста на следующую строку */
word-wrap: break-word; /* Переносим слова, если они выходят за границы */
}
}
</style>
</head>
<body>
<div class="award-card">
<h2>Награды персонажа</h2>
<div class="awards">
<div class="award-item">
<div class="award-icon-container">
<img src="https://forumstatic.ru/files/001a/74/14/44576.png" alt="Медаль за храбрость" class="award-icon">
</div>
<span class="award-name">Храбрость</span>
<div class="tooltip">За проявленную храбрость в бою</div>
</div>
<div class="award-item">
<div class="award-icon-container">
<img src="https://forumupload.ru/uploads/0013/66/99/2/90257.png" alt="Орден чести" class="award-icon">
</div>
<span class="award-name">Честь</span>
<div class="tooltip">Награда за соблюдение кодекса чести</div>
</div>
<div class="award-item">
<div class="award-icon-container">
<img src="https://forumstatic.ru/files/001a/74/14/97862.png" alt="Знак отличия" class="award-icon">
</div>
<span class="award-name">Отличие</span>
<div class="tooltip">Выдан за особые заслуги</div>
</div>
<div class="award-item">
<div class="award-icon-container">
<img src="https://forumstatic.ru/files/001a/74/14/62576.png" alt="Почетная грамота" class="award-icon">
</div>
<span class="award-name">Грамота</span>
<div class="tooltip">Получена за отличные результаты</div>
</div>
<div class="award-item">
<div class="award-icon-container">
<img src="https://static.wikia.nocookie.net/fortnite/images/5/50/Scroll_-_Back_Bling_-_Fortnite.png" alt="Приз зрительских симпатий" class="award-icon">
</div>
<span class="award-name">Приз</span>
<div class="tooltip">Приз, выбранный зрителями</div>
</div>
</div>
</div>
</body>
</html>
[/html]